本文记录一下在刷leetcode算法题过程中积累的一些技巧和方法:
Java本地调试
- 以
81.搜索旋转排序数组-ii.java
为例,其代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
|
class Solution { public boolean search(int[] nums, int target) { int first = 0; int last = nums.length; int mid; while (first != last) { mid = (first+last)>>>1; if (nums[mid] == target) return true; if (nums[first] < nums[mid]) { if (nums[first] <= target && target < nums[mid]) { last = mid; } else { first = mid + 1; } } else if (nums[first] > nums[mid]) { if (nums[mid] < target && target <= nums[last - 1]) { first = mid + 1; } else { last = mid; } } else { first++; } } return false; } }
|
- 在上述文件夹下新键
Test
类即Test.java
文件用于测试,并将上述Solution
类名改为S
避免冲突:
1 2 3 4 5 6 7 8
| public class Test { public static void main(String[] args) { S s1 = new S(); int[] nums = new int[]{1, 0, 1, 1, 1}; int target = 0; System.out.println(s1.search(nums, target)); } }
|
- 以上述
S
类和Test
类为例,由于在debug时实在本地查找相应的.class
文件,所以需要在本地编译得到S.class
和Test.class
后再调试(下述S.class
可以由其他文件名的.java
文件编译得到,如18.四数之和.java
:

- 在下述位置打断点并点击
Debug
按钮进入调试:
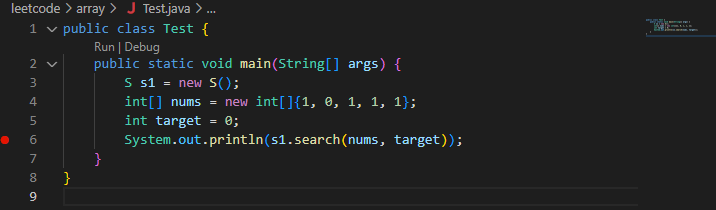
- 注意需要安装
Extension Pack for Java
以及Code Runner
等插件,系统里需要安装java jdk。
参考资料:
- 免费 Leetcode Debug?教你用VSCode优雅的刷算法题!!!_哔哩哔哩_bilibili
C++本地调试
- 同样以
81.cpp
为例,注意进行C++调试时.cpp
文件和路径名中不要含有中文。81.cpp
代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
|
#include <bits/stdc++.h> using namespace std;
class Solution { public: bool search(vector<int>& nums, int target) { int first =0; int last = nums.size(); int mid; while(first!=last){ mid = first + (last-first)/2; if(nums[mid]==target) return true; if(nums[first]<nums[mid]){ if(nums[first]<=target && target<nums[mid]){ last=mid; }else{ first=mid+1; } }else if(nums[first]>nums[mid]){ if(nums[mid]<target && target<=nums[last-1]){ first=mid+1; }else{ last=mid; } }else{ first++; } } return false; } };
|
1 2 3 4 5 6 7
| int main(){ vector<int> nums; nums={1, 0, 1, 1, 1}; int target = 0; Solution s; cout << s.search(nums, target) << endl; }
|
- 在下述位置打断点并点击右上角
Debug C/C++ File
进行调试:
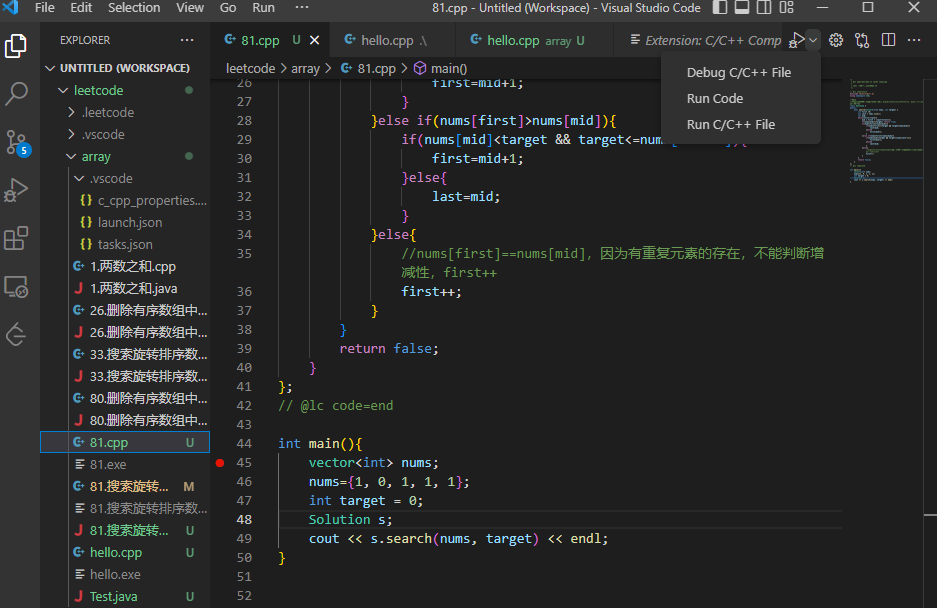
- 注意在调试之前,需要配置好C++环境(即
.vscode
文件夹),并安装C/C++
和Code Runner
等插件。
参考资料:
leetcode刷题本地调试模板(C++) – SAquariusの梦想屋
C++ vector<int>&nums
用法总结
康托展开和逆康托展开
参考资料:
- 康托展开和逆康托展开_wbin233的博客-CSDN博客_逆康托展开